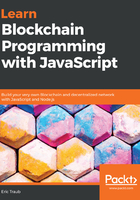
An explanation of the JavaScript constructor function
Becoming familiar with the constructor function is important as we'll be using it to build our blockchain data structure. By now, you must be wondering what a constructor function is and what it actually does.
A constructor function is simply a function that creates an object class and allows you to easily create multiple instances of that particular class. What this actually means is that the constructor function allows you to create a lot of objects very quickly. All of these objects that are created will have the same properties and functionalities because they are all part of the same class. Now, all of this might seem a little bit confusing when you hear it for the first time, but don't worry — we'll try to understand what a constructor function is with the help of an example.
Let's take Facebook, for example. Facebook has over one-and-a half billion users, which are all objects of the same class and have similar properties such as name, email, password, birthday, and so on. For our example, let's assume that we are building the Facebook website and want to create a bunch of different users for it. Let's do this by creating a User constructor function.
To learn and explore the constructor functions, let's use the Google Chrome console. We can access the console by going to Google Chrome and simply pressing command + option + J for Mac users and Ctrl + Shift + I for Windows users. Alternatively, we can simply go to the menu option, go to More Tools, and then select the Developer Tools option, as shown in the following screenshot:
Following the aforementioned steps will open the console for you, as shown in the following screenshot:
The constructor function that we'll be coding in this example will allow us to create multiple users or multiple user objects that will have the same properties and functionalities. The code to create this User constructor function begins by defining it as follows:
function User() {
}
Inside of the parentheses, (), let's pass the properties that we want each of our User objects to have. We're going to pass properties such as firstName, lastName, age, and gender because we want all of our user objects to have these components.
We then assign these parameters to our User objects by using the this keyword, as shown in the following block of code:
This is how we define the constructor function in JavaScript. Now, reading through the preceding code block, you might be wondering what we did and what the this keyword is all about.
We're going to use this constructor function to create a lot of user objects. The this keyword is simply referring to each of the user objects that we're going to create. This might all seem a bit overwhelming right now, but let's run through a couple of examples and try to gain more clarity on it.
Let's begin using our User constructor function. To make some User objects, also known as User instances, follow these steps:
- The first user that we are going to create – let's call it user1 – will be defined as follows:
var user1 = new User('John','Smith',26,'male');
In the preceding code, you may have noticed that we used the new keyword to invoke our constructor function and make a user object, which is how we get our constructor function to work.
- Then press Enter, and user1 is in the system. Now, if we type user1 in the console, we'll be able to see what we just created in the previous step:
In the preceding output screenshot, we can see that user1 is an object of the User class. We can also see that user1 has a firstName of John, a lastName of Smith, an age of 26, and gender of male because these are the parameters that we passed into the constructor function.
- For clarity, try adding one more user. This time, we'll create another user called user200 and pass in into the new User ( ) function with the user's properties, such as a first name of Jill, a last name of Robinson, an age of 25, and a female gender:
var user200 = new User('Jill', 'Robinson', 25, 'female');
- By pressing Enter, our new user200 will be in the system. Now, if we type user200 into the console and press Enter, we'll see the following output:
In the preceding output, we can see that user200 is an object of the User class, just like user1, and that she has a first name of Jill, a last name of Robinson, an age of 25, and a female gender because these were the parameters that we passed into our constructor function.
Now, you might be wondering how all of these properties that we mentioned got assigned correctly. This was all due to the this keyword that we mentioned earlier. When we create our constructor function, we use the this keyword to assign properties. When it comes to a constructor function, the this keyword does not refer to the function that it is in – in our case, the User function. Instead, this refers to the object that will be created by the constructor function.
This signifies that if we use the constructor function to create an object, we must make sure that the property and their objects are first name, last name, age, and gender, or whenever you make your constructor function, set the firstName property as equal to the firstName parameter that is passed in, and do the same for the rest of the properties.
This is how a constructor function works and how the this keyword plays an important role in the constructor function.