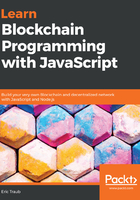
Building the createNewBlock method
Let's continue with building our blockchain data structure. After defining our constructor function in the previous section, the next thing that we want to do with our constructor function is to place a method in our Blockchain function. This method that we are going to create will be called createNewBlock. As its name suggests, this method will create a new block for us. Let's follow the below mentioned steps to build the method:
- The createNewBlock method will be defined as follows:
Blockchain.prototype.createNewBlock = function () {
}
- Now we've got this createNewBlock method on our blockchain prototype object. This method will take the three parameters, as highlighted in the following line of code:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
}
We'll learn in depth about these three parameters in further sections, so don't worry if you're not familiar with them.
- Now, the next thing that we want to do inside of our createNewBlock method is to create a newBlock object. Let's define this as follows:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
};
}
This newBlock object is going to be a new block inside of our BlockChain, so all of the data is going to be stored inside of this block. This newBlock object is a pretty important part of our blockchain.
- Next, on the newBlock object, we're going to have an index property. This index value will basically be the block number. It will describe what number of block the newBlock is in our chain (for example, it may be the first block):
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
index: this.chain.length + 1,
};
}
- Our next property is going to be a timestamp, because we want to know when the block was created:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
index: this.chain.length + 1,
timestamp: Date.now(),
};
}
- Then, the next property we will add will be for the transactions. When we create a new block, we'll want to put all of the new transactions or the pending transactions that have just been created into the new block so that they're inside of our blockchain and can never be changed:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
index: this.chain.length + 1,
timestamp: Date.now(),
transactions: this.newTransactions,
};
}
The preceding highlighted line of code states that all of the transactions in the block should be the new transactions that are waiting to be placed into a block.
- The next property that we are going to have on our block is a nonce, and this will be equal to the nonce parameter that we passed into our function earlier:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
index: this.chain.length + 1,
timestamp: Date.now(),
transactions: this.newTransactions,
nonce: nonce,
};
}
Now, you might be wondering what a nonce is. Basically, a nonce comes from a proof of work. In our case, this is simply any number; it doesn't matter which. This nonce is pretty much proof that we've created this new block in a legitimate way by using a proofOfWork method.
- The next property is going to be a hash:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
index: this.chain.length + 1,
timestamp: Date.now(),
transactions: this.newTransactions,
nonce: nonce,
hash: hash,
};
}
Basically, this hash will be the data from our newBlock. What's going to happen is we're going to pass our transactions or our newTransactions into a hashing function. What this means is that all of our transactions are going to be compressed into a single string of code, which will be our hash.
- Finally, our last property on our newBlock will be our previousBlockHash:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
index: this.chain.length + 1,
timestamp: Date.now(),
transactions: this.newTransactions,
nonce: nonce,
hash: hash,
previousBlockHash: previousBlockHash,
};
}
This previousBlockHash property is very similar to our hash property, except our hash property deals with the data from our current block hashed into a string, and the previousBlockHash property deals with the data from our previous block or the previous block to the current block hashed into a string.
So, hash and previousBlockHash are both hashes. The only difference is that the hash property deals with the data of the current block, and, the previousBlockHash property deals with the hashing of the data of the previous block. This is how you create a new block, and this is what every block in our blockchain will look like.
- Continuing with our createNewBlock method, the next thing that we want to do is set this.newTransaction as equal to an empty array, as follows:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
index: this.chain.length + 1,
timestamp: Date.now(),
transactions: this.newTransactions,
nonce: nonce,
hash: hash,
previousBlockHash: previousBlockHash,
};
this.newTransaction = [];
}
We do this because, once we create our new block, we are putting all of the new transactions into the newBlock. Therefore, we want to clear out the entire new transactions array so that we can start over for the next block.
- Next, what we simply want to do is take the new block that we've created and push it into our chain, and then we're going to return the newBlock:
Blockchain.prototype.createNewBlock = function (nonce, previousBlockHash, hash) {
const newBlock = {
index: this.chain.length + 1,
timestamp: Date.now(),
transactions: this.newTransaction,
nonce: nonce,
hash: hash,
previousBlockHash: previousBlockHash,
};
this.newTransaction = [];
this.chain.push(newBlock);
return newBlock;
}
By adding these last two lines of code, our createNewBlock method is ready. Basically, what this method does on a high level is it creates a new block. Inside of this block, we have our transactions and the new transactions that have been created since our last block was mined. After we've created a new block, let's clear out the new transactions, push the new block into our chain, and simply return our new block.