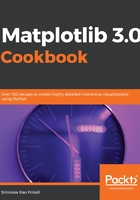
上QQ阅读APP看书,第一时间看更新
There's more...
Here is another example of using a mix of centimeters and inches on the x and y axis. We have four bar plots with the same input data, plotted on a 2 x 2 grid.
Import the required libraries:
import numpy as np
from basic_units import cm, inch
import matplotlib.pyplot as plt
The following code plots the figure with four bar plots in a 2 x 2 grid:
- Define the data and the units of measurement:
cms = cm * np.arange(0, 21, 3)
bottom = 0 * cm
width = 0.8 * cm
- Define the figure and its layout:
fig, axs = plt.subplots(2, 2)
- Plot a bar chart on the first axes, and set xticks in centimeters:
axs[0, 0].bar(cms, cms, bottom=bottom)
axs[0, 0].set_xticks(cm * np.arange(0, 21, 3))
- Plot a bar chart on the second axes with the x axis in centimeters and the y axis in inches:
axs[0, 1].bar(cms, cms, bottom=bottom, width=width, xunits=cm,
yunits=inch)
axs[0, 1].set_xticks(cm * np.arange(0, 21, 3))
- Plot a bar chart on the third axes with the x axis in inches and the y axis in centimeters:
axs[1, 0].bar(cms, cms, bottom=bottom, width=width, xunits=inch,
yunits=cm)
axs[1, 0].set_xticks(cm * np.arange(0, 21, 3))
axs[1, 0].set_xlim(2, 6)
- Plot a bar chart on the fourth axes with both the x and the y axis in inches:
axs[1, 1].bar(cms, cms, bottom=bottom, width=width, xunits=inch,
yunits=inch)
axs[1, 1].set_xticks(cm * np.arange(0, 21, 3))
axs[1, 1].set_xlim(2 * cm, 6 * cm)
- Adjust the space between the plots and display the figure on the screen:
fig.tight_layout()
plt.show()
Here is the explanation of how it works:
- Prepare the input data for both the x and the y coordinates, set the bottom offset for the bars, and the width of the bar.
- fig, axs = plt.subplots(2, 2) defines the figure layout in a 2 x 2 grid.
- axs[0, 0].bar(cms, cms, bottom=bottom) plots a bar plot on axs[0, 0] and the input data is in centimeters. axs[0, 0].set_xticks(cm * np.arange(0, 21, 3)) sets the tick lines on the x axis so that they are aligned with the bars.
- The bar plot on axs[0, 1] is exactly same as axs[0, 0], except that the units for the y axis changed from centimeters to inches, which is visible on the plot.
- The bar plot on axs[1, 0] is again similar to the one on axs[0, 0], but the units on the x axis changed to inches, and axs[1, 0].set_xlim(2, 6) sets the x axis limits to be (2, 6). Here, only scalars are used to set the limits, without any unit of measurement, which means whatever the x axis unit of measurement is, the same would be applicable for the limits, which in this case range from two to six inches.
- The bar plot on axs[1,1] uses inches as the unit of measurement for both the x and the y axis and sets the x axis limits in centimeters (2, 6), which will be converted to inches in alignment with the unit of measurement for the x axis. So it would be converted to inches, which in this case translates to 0.79 to 2.362 inches.
You should see a figure like the following: