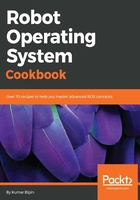
上QQ阅读APP看书,第一时间看更新
Using a plugin
In the previous section, we successfully created and exported a few of the RegularPolygon plugins. To discuss how to use them, we will create src/polygon_loader.cpp with the following contents:
#include <pluginlib/class_loader.h> #include <pluginlib_tutorials/polygon_base.h> int main(int argc, char** argv) { pluginlib::ClassLoader<polygon_base::RegularPolygon> poly_loader("pluginlib_tutorials", "polygon_base::RegularPolygon"); try { boost::shared_ptr<polygon_base::RegularPolygon> triangle = poly_loader.createInstance("polygon_plugins::Triangle"); triangle->initialize(10.0); boost::shared_ptr<polygon_base::RegularPolygon> square = poly_loader.createInstance("polygon_plugins::Square"); square->initialize(10.0); ROS_INFO("Triangle area: %.2f", triangle->area()); ROS_INFO("Square area: %.2f", square->area()); } catch(pluginlib::PluginlibException& ex) { ROS_ERROR("The plugin failed to load for some reason. Error: %s", ex.what()); } return 0; }
The preceding source code loads the instance of Triangle and Square plugins belonging to RegularPolygon plugins and calculates the area. The catch block of code displays the reasons for failure if the try block fails.