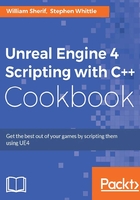
Unmanaged memory – using malloc( )/free( )
The basic way to allocate memory for your computer program in C (and C++) is by using malloc()
. malloc()
designates a block of the computer system's memory for your program's use. Once your program is using a segment of memory, no other program can use or access that segment of memory. An attempt to access a segment of memory not allocated to your program will generate a "segmentation fault", and represents an illegal operation on most systems.
How to do it...
Let's look at an example code that allocates a pointer variable i
, then assigns memory to it using malloc()
. We allocate a single integer behind an int*
pointer. After allocation, we store a value inside int
, using the dereferencing operator *
:
// CREATING AND ALLOCATING MEMORY FOR AN INT VARIABLE i int* i; // Declare a pointer variable i i = ( int* )malloc( sizeof( int ) ); // Allocates system memory *i = 0; // Assign the value 0 into variable i printf( "i contains %d", *i ); // Use the variable i, ensuring to // use dereferencing operator * during use // RELEASING MEMORY OCCUPIED BY i TO THE SYSTEM free( i ); // When we're done using i, we free the memory // allocated for it back to the system. i = 0;// Set the pointer's reference to address 0
How it works…
The preceding code does what is shown in the diagram that follows:
- The first line creates an
int*
pointer variablei
, which starts as a dangling pointer referring to a segment of memory that probably won't be valid for your program to reference. - In the second diagram, we use a
malloc()
call to initialize the variablei
to point to a segment of memory precisely the size of anint
variable, which will be valid for your program to refer to. - We then initialize the contents of that memory segment to the value
0
using the command*i = 0;
.Tip
Note the difference between assignment to a pointer variable (
i =
), which tells the pointer what memory address to refer to, and assignment to what is inside the memory address that the pointer variable refers to (*i =
).
When the memory in the variable i
needs to be released back to the system, we do so using a free()
deallocation call, as shown in the following diagram. i
is then assigned to point to memory address 0
, (diagrammed by the electrical grounding symbol reference ).

The reason we set the variable i
to point to the NULL
reference is to make it clear that the variable i
does not refer to a valid segment of memory.