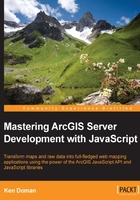
Layers
No map is complete without data to show. Data is added to the map through layers. Layers refer to data sources that include geographic, symbolic, and even tabular data. The ArcGIS API for JavaScript contains a number of modules for interpreting different kinds of common geographic data sources. Some of the layer modules work with data provided by ArcGIS Server, and others can display data from other sources, such as KML, Web Map Service (WMS), and CSV files.
Common ArcGIS Server layers
ArcGIS Server provides map layer data through Map Services. Map Services provide data published from an ArcMap map document (.mxd
). The visual map data, legend, and underlying data tables are served through ArcGIS Server, and can be consumed through the browser when the layer data source is loaded into the map. Different layer types have different functionalities. We will review those layers in the following sections.
An ArcGISDynamicMapServiceLayer
is the default type of map published through the ArcGIS Server. It is a dynamic map, meaning that its content is updated whenever the map is refreshed. Because it is dynamic, the data can also be reprojected to fit on another map layer that is not in the same spatial reference.
require(["esri/map", "esri/layers/ArcGISDynamicMapServiceLayer"], function (Map, ArcGISDynamicMapServiceLayer) { … var map = new Map("mapdiv", {…}); var layer = new ArcGISDynamicMapServiceLayer ("http://serv.er/arcgis/rest/services/MyDynamicLayer/mapserver"); map.addLayer(layer); });
Because the data within an ArcGISDynamicMapServiceLayer
is dynamic, ArcGIS Server redraws what the layer should look like every time the map looks at the data from a different location and zoom level. If this layer contains lots of labels, annotation, complicated graphics, or raster data (like aerial imagery, for instance), ArcGIS Server will require more system resources to render the map layer. This will lead to noticeably slower performance. Other services, like tiled map services, are recommended for that kind of data.
ArcGIS Server allows map services to be tiled. An ArcGISTiledMapServiceLayer
has its content already drawn into images at scales defined when the map service is published. These images are stored on a hard drive for quick retrieval. The benefit is that these pre-rendered tiles are served quickly and with little effort on the part of the server. More map services can be run from the same machine with little effect on performance. See an example in the following code snippet:
require(["esri/map", "esri/layers/ArcGISTiledMapServiceLayer"], function (Map, ArcGISTiledMapServiceLayer) { … var map = new Map("mapdiv", {…}); var layer = new ArcGISTiledMapServiceLayer ("http://serv.er/arcgis/rest/services/MyTiledLayer/mapserver"); map.addLayer(layer); });
Tiled map services have some disadvantages. First, the data displayed through tiled map services doesn't change until the tiles are rebuilt. If a park boundary changes in the data, but the tiles aren't rebuilt, the service will still show the old boundary. Second, tiled map services restrict the map to specific zoom scales, with no easy way to view the map in between zoom scales. Third, if another tiled map is stacked on top of the first one, it not only has to be in the same spatial reference, but also the zoom scales have to overlap exactly within overlapping scale ranges. That means that a map built with scales of 1:500 and 1:1000 can be loaded with a map built with scales of 1:1000 and 1:2000, but a tiled layer with scales 1:750, 1:1500, and 1:3000 will not be seen.
Tip
You cannot load a dynamic map service layer as an ArcGISTiledMapServiceLayer
, or else it will throw an error. However, a tiled map service can be loaded as an ArcGISDynamicMapServiceLayer
. You lose performance when zooming and panning, but it will help you view scales in between the tiled scales.
We've seen ArcGIS layers that provide image data. Let's turn our attention to layers that handle more vector data.
GraphicsLayers and FeatureLayers
Sometimes you don't want to work with representative images of maps. Sometimes you want to work with the shape of your data directly. That's where GraphicsLayers
and FeatureLayers
come in. These layers render vector graphics directly in your browser. You can click on them, modify them, and even add data to an ArcGIS service through them. Let's look a little closer at them.
GraphicsLayers
provide a simple way to add custom vector graphics to your map. You can manage the graphics by calling add()
and remove()
methods on those graphics. You can also search through the list of graphics on the map by searching through the GraphicsLayer.graphics
array.
As a side note, the map object has a built-in GraphicsLayer
. You can access that GraphicsLayer
through the map.graphics
property.
FeatureLayers
are specialized layers built on a GraphicsLayer
that provide much more functionality. They have the custom vector graphics of a GraphicsLayer
, plus they have an ArcGIS Server data source to populate the data. You can query for data by either location or attributes, add it to the map using custom graphics, and control popup content and styling. You can even edit data on your server.
Unlike ArcGIS Tiled and dynamic layers, which can mash up multiple layers at once, each FeatureLayer
can only work with one map service layer. FeatureLayers
can work with individual layers in a map service, or special editable map services called Feature Services. Feature Services provide more details about the map layer, including endpoints for querying results. If ArcGIS Server is configured to allow editing on the features, and the map service is published to allow editing, FeatureLayers
that consume these services will let you modify both shape and tabular data.
Because FeatureLayers
query data from ArcGIS Server, they are limited in the number of features they can return. Typically, ArcGIS Server limits the number of results returned in a query to one or two thousand, depending on the version. Server settings can be altered to show more results, but more FeatureLayer
graphics on the map mean more memory is consumed, and the app may be slow and unresponsive. Careful thought must be put into using the FeatureLayer
with your data.
One way that the FeatureLayer
refines the number of features returned is by setting its mode. The FeatureLayer
has three primary modes of feature selection:
MODE_ONDEMAND
: Only features that fit within the map extent, time extent, and/or definition query are downloaded. This is the most common way of interacting with features visible within the map extent.MODE_SELECTION
: Only features that are selected by interacting with the map (by click, touch, or query, for instance) are downloaded and their data is shown on the map.MODE_SNAPSHOT
: All features are initially downloaded into memory. This method is popular for smaller datasets, and requires fewer server calls.MODE_AUTO
: It automatically picks betweenMODE_ONDEMAND
andMODE_SNAPSHOT
and is fairly new to the ArcGIS API for JavaScript, depending on the size and content of the data.
We will look into FeatureLayers
more closely in Chapter 5, Editing Map Data when we discuss editing.
Other layer types
The ArcGIS API for JavaScript works well with data published through ArcGIS Server. But not all geographic data available in the world is published through ArcGIS Server. The API has a number of other layer types that can consume these different data sources. Some of the more common types are as follows:
KMLLayer
: Services written with the Keyhole Markup Language (KML). KML was made famous by Google Earth. The format uses the markup to store and symbolize geographic data. Many services publish KML data, including the National Oceanic and Atmospheric Administration (NOAA).CSVLayer
: A fairly recent addition, it transforms a comma-delimited text file into points on the map. To show the data on a map, theCSVLayer
expects a latitude and longitude column to show the coordinates in decimal places. This tool, combined with HTML5's drag-and-drop API, could make it possible for you to map any compliant data you toss into a.csv
file.WMSLayer
andWMTSLayer
: Web Map Services (WMS) and Web Map Tiled Services (WMTS) published using the Open Geospatial Consortium (OGC) compliant standards.Note
Please note that if you load the layer using just the URL of the OGC Web Map Service, you'll need to use a proxy (see Chapter 1, Your First Mapping Application). This is because the module first requests
GetCapabilities
on the layer to grab metadata from the map service. This request requires a proxy. However, if you load theWMSLayer
with an optionalresourceInfo
parameter, that automatically describes the service for you, and the proxy will not be necessary.StreamLayer
: A layer displaying live streaming data from an ArcGIS GeoEvent Processor Extension.StreamLayer
takes advantage of the latest in HTML5 WebSockets, which provide real-time updates from the server to the client. You can view Twitter responses to specific events, or real-time locations of emergency response vehicles with tracking devices.
Note
Note that most up-to-date modern browsers support WebSockets technology, but older browsers typically do not. You can visit http://www.websocket.org/echo.html to see if your browser supports WebSockets.