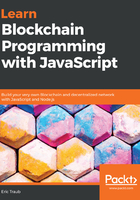
Testing the createNewTransaction method
Let's test the createNewTransaction method that we created in the previous section. Just as a heads up: this section is going to be a lot of fun, as here you will really start to understand how powerful a blockchain can be and how blocks and transactions work with each other. You'll also get to learn how the transactions are recorded in the blockchain. So let's get started:
- We're going to test our createNewTransaction method in our test.js file. In this file, we've already required our blockchain.js file and have made a new instance of our Blockchain called bitcoin, which we are logging out at the end of our file. Take a look at the following screenshot for a quick review:
- Now, the first thing that we're going to do in our test.js file is create a new block using our createNewBlock method, similarly to what we did in the Testing the createNewBlock method section. Type in the following into your test.js file:
bitcoin.createNewBlock(789457,'OIUOEDJETH8754DHKD','78SHNEG45DER56');
- Next, what we want to do is create some new transactions to test our createNewTransaction method. This createNewTransaction method takes in three parameters, such as amount, a sender, and the recipient. Let's add this transaction data to our test case:
bitcoin.createNewTransaction(100,'ALEXHT845SJ5TKCJ2','JENN5BG5DF6HT8NG9');
In the preceding line of code, we've set the amount of the transaction to 100 and the sender and recipient's address to some random hash numbers.
Now, let's just quickly summarize what we have done so far in our test case. Take a look at the following code block:
const Blockchain = require('./blockchain');
const bitcoin = new Blockchain();
bitcoin.createNewBlock(789457,'OIUOEDJETH8754DHKD','78SHNEG45DER56');
bitcoin.createNewTransaction(100,'ALEXHT845SJ5TKCJ2','JENN5BG5DF6HT8NG9');
console.log(bitcoin);
In the preceding code, we first required the bitcoin blockchain, and then we created a new block. After that, we created a new transaction, and then we logged out the bitcoin blockchain.
When we run this test.js file, we should expect to see our bitcoin blockchain, which should have one block in the chain as well as one transaction in the pendingTransactions array because we have not mined or created a new block after creating the transaction. Let's save this file and run it to see what we get.
- Now go to your terminal window, type in the following command, and then press Enter:
node dev/test.js
We get to observe the bitcoin blockchain on the terminal window, as shown in the following screenshot:
In the output on your window and in the preceding screenshot, you can observe our chain, which has the one block that we created. In our pendingTransactions array, we have one pending transaction, which is the transaction we created in the test case. Looking at the output of the test, we can conclude that, so far, our createNewTransaction method works fine.