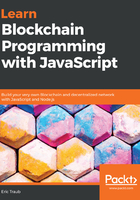
Adding a pending transaction to our blockchain
Now let's try to understand how we can get the pendingTransaction into our actual chain up here. The way we do that is by mining a new block or by creating a new block. Let's do that now:
- After we create the newTransaction, let's create a new block using the createNewBlock method, as highlighted in the following code:
const Blockchain = require('./blockchain');
const bitcoin = new Blockchain();
bitcoin.createNewBlock(789457,'OIUOEDJETH8754DHKD','78SHNEG45DER56');
bitcoin.createNewTransaction(100,'ALEXHT845SJ5TKCJ2','JENN5BG5DF6HT8NG9');
bitcoin.createNewBlock(548764,'AKMC875E6S1RS9','WPLS214R7T6SJ3G2');
console.log(bitcoin);
What we have done is created a block, created a transaction, and then mined a new block. Now the transaction that we created should show up in our second block because we mined a block after we created a transaction.
- Now save the file and run the test again. Let's see what we get from this. Go to your terminal and again type in the node dev/test.js command and press Enter. You will get to observe the output that is shown in the following screenshot:
Here, we have our entire blockchain again, which has two blocks in it because we mined two blocks. The chain has our first block (index: 1), which has no transactions and has our second block (index: 2), in which, if you look at our transactions, it says that there is an Array that has items in it versus a first block's transaction array, which has no items in it.
- Now take a closer look at the second block's transaction array. We should expect to see the transaction that we had created previously. Let's make the following highlighted modification to our test case:
const Blockchain = require('./blockchain');
const bitcoin = new Blockchain();
bitcoin.createNewBlock(789457,'OIUOEDJETH8754DHKD','78SHNEG45DER56');
bitcoin.createNewTransaction(100,'ALEXHT845SJ5TKCJ2','JENN5BG5DF6HT8NG9');
bitcoin.createNewBlock(548764,'AKMC875E6S1RS9','WPLS214R7T6SJ3G2');
console.log(bitcoin.chain[1]);
- In this modification, we just log out of the second block in our chain. [1] in the code defines the position of the second block. Save this file and run it. In the output, you can observe that we are simply logging out of the second block in our chain and you can see that, for transactions, it has an array with one object in it. Check out the following screenshot:
This object is the transaction that we created in our test. What we did here was just create a transaction and then mine it by creating a new block or mining a new block, which now has our transaction in it.
Now, let's carry out a couple more examples to help clarify what is happening here. Let's take the createNewTransaction method and duplicate it three more times after our createNewBlock method. Make the modifications to the amounts as you wish to.
What's happening over here is that, from the top, we are first creating a block and then creating a transaction. We are then creating or mining a new block, so we should have one block with no transactions and another block with one transaction in it. After we create our second block, we are creating three more new transactions. At this point, all three of these new transactions should be in our pendingTransactions array because we are not creating a new block after we create these three transactions. Lastly, we log out of our bitcoin blockchain again. Your test should now look similar to the following:
const Blockchain = require('./blockchain');
const bitcoin = new Blockchain();
bitcoin.createNewBlock(789457,'OIUOEDJETH8754DHKD','78SHNEG45DER56');
bitcoin.createNewTransaction(100,'ALEXHT845SJ5TKCJ2','JENN5BG5DF6HT8NG9');
bitcoin.createNewBlock(548764,'AKMC875E6S1RS9','WPLS214R7T6SJ3G2');
bitcoin.createNewTransaction(50,'ALEXHT845SJ5TKCJ2','JENN5BG5DF6HT8NG9');
bitcoin.createNewTransaction(200,'ALEXHT845SJ5TKCJ2','JENN5BG5DF6HT8NG9');
bitcoin.createNewTransaction(300,'ALEXHT845SJ5TKCJ2','JENN5BG5DF6HT8NG9');
console.log(bitcoin);
Now, if we save the file and run it, we should have two blocks in our chain, and we should also have three transactions in the pendingTransactions array. Let's see what we get here. You will get to observe the following output on your screen:
In the preceding screenshot, you can observe that we have our blockchain. In this chain, we have two blocks, just like we expected to have, and in our pendingTransactions array, we have three transactions, which are the three transactions that we had created in our test file.
What we have to do next is get these pending transactions into our chain. For that, let's mine another block. Just copy and paste the creatNewBlock method after the three transactions that we created and make modifications to its parameters as you wish to. When we run the test now, the three transactions that are pending should appear in our new block. Let's save the file and run the test. You will get to observe the following output:
So, we have our blockchain, which has three blocks in it. Our pendingTransactions array is currently empty, but where did those three transactions go? As it turns out, they should be in the last block that we created, which is the index: 3 block. Inside of this third block we have our transactions, which should be the three transactions we just created. Let's take a deeper look at this by making a tiny modification to the last line of our test code, which is console.log(bitcoin.chain[2]); . The value 2 here specifies the third block in the chain. Let's save this modification and run the test again. You will get to see the third block in the chain:
In the transaction's array, you can see that we have all three of the transactions that we created. So, this is how our createNewTransaction and createNewBlock methods work with each other.