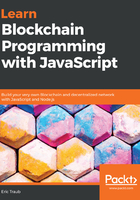
The hashBlock method
Let's build up our hashBlock method. Inside of this method, we want to use SHA256 hashing to hash our block data. Follow the below mentioned steps:
- To use an SHA256 hashing function, import it as an npm library. To do that, go to Google and type SHA256 into the search bar, or visit https://www.npmjs.com/package/sha256. On this website, you will get to see the command that we need to type into our terminal to install SHA256. We'll have to type in the following command in our terminal:
npm i sha 256--save
- After doing this, press Enter. The --save in the following command will save this library as a dependency for us. Now, inside of our blockchain file structure, you may see that the node_modules folder has appeared. Inside of this folder is where our SHA256 library and all other dependencies have been downloaded.
- To use this SHA256 library, we will have to import the library to our code so that we can use it. At the very start of our code, type the following line:
const sha256 = require('sha256');
The preceding line of code specifies that we have an SHA256 hashing function stored as the variable SHA256 in the blockchain.js file. By importing it, we can use that inside of our hashBlock method.
- Now, the first thing that we want to do in our hashBlock method is to change the parameters that it takes. We're going replace the blockData parameter with previousBlockHash, currentBlockData, and nonce:
Blockchain.prototype.hashBlock = function(previousBlockHash, currentBlockData, nonce) {
}
These three parameters will be the data that we are going to be hashing inside of our hashBlock method. All of this data will come from a single block in our chain and we're going to hash this data, which is essentially hashing a block. We are then going to get a hashed string in return.
- The first thing that we want to do is change all of these pieces of data into a single string, so add the following line of code to our hashBlock method:
const dataAsString = previousBlockHash + nonce.tostring()+ JSON.stringify( currentBlockData);
In the preceding code, previousBlockHash is already a string. Our nonce is a number, so we're going to change that to a string with toString. Furthermore, our currentBlockData is going to be an object, an array of our transactions, or some kind of JSON data. It will either be an array or an object, and JSON.stringify will simply turn that data (as well as any object or array) into a string. Once this whole line is run, we will simply have all of the data that we passed to be concatenated into a single string.
- Now, the next thing that we want to do is create our hash, as follows:
const hash = sha256(dataAsString);
This is how we create a hash from our block or all of the block data that we have passed into our function.
- The last thing that we want to do is simply return the hash, so before we complete this method, add the following:
return hash;
This is how our hashBlock method will work. In the following section, we will test this method to see if it works perfectly.