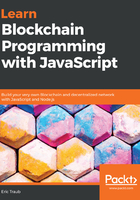
Testing the hashBlock method
Let's test our hashBlock method in the test.js file. Similar to what we did in the previous sections, in our test.js file, we should be importing our blockchain data structure, creating a new instance of our blockchain, and naming it bitcoin. Now, let's test our hashBlock method:
- For that, type in the following highlighted line of code into our test.js file:
const Blockchain = require ('./blockchain');
const bitcoin = new Blockchain ();
bitcoin.hashBlock();
- Our hashBlock method requires three parameters: a previousBlockHash, currentBlockData, and the nonce. Let's define these variables above the part where we're calling our hashBlock method. We'll begin by defining the previousBlockHash:
const previousBlockHash = '87765DA6CCF0668238C1D27C35692E11';
For now, this random string/hash data will act as input for our previousBlockHash.
- Next, we create the currentBlockData variable. This currentBlockData will simply be an array of all of the transactions that will be present in this block. We're simply going to use the transactions in this block as our currentBlockData, so in this array, we will have to make a couple of transaction objects, as follows:
const currentBlockData = [
{
amount: 10,
sender: 'B4CEE9C0E5CD571',
recipient: '3A3F6E462D48E9',
}
]
- Next, duplicate this transaction object at least three times to make a couple more transaction objects in the array and then make modifications to the data as you wish, aiming to change the amount and the sender's and recipient's addresses. This will make our currentBlockData an array that holds three transactions.
- Finally, we have to assign the nonce value in our hashBlock method:
const nonce = 100;
- After defining these variables, we call the hashBlock method and pass the previousBlockHash and currentBlockData parameters, as well as the nonce:
bitcoin.hashBlock(previousBlockHash, currentBlockData, nonce );
- Furthermore, let's try to push the results onto the terminal window so that we can observe it. To do this, we'll have to make a tiny modification to our preceding code:
console.log(bitcoin.hashBlock(previousBlockHash, currentBlockData, nonce));
In this test case, we are calling our hashBlock method with all of the correct parameters. When we run this file, we should get to observe the hash on the terminal window.
- Now save this test.js file and run it to check whether or not we get the output that we expect it to be.
- Go to your terminal window and type in the node dev/test.js command, and let's observe what results we get. You will get to observe the similar resulting hash as output of our hashBlock method as follows:
It looks like our hashBlock method is working pretty well.
- Try to explore this hashBlock method a little more. As explained in the previous section, if we change some of the data that we are passing into the hashBlock method, it will result in completely changing the hash that we returned as output.
- Now try to test this feature of hashing data by changing a letter in any of the sender's or recipient's address. Then save the file and run it by using node dev/test.js again. You will get to observe a totally different hash data as output, as follows:
In the preceding screenshot, you can observe both the hash data and the differences between them.
Now, if we revert the change that we made to the sender's or recipient's address and run our hash method again, we'll get to observe the same hash that we originally got. This is because we're passing in the same data that we did the first time around. You can try experimenting with the data and try observing the output to explore the hashBlock method further.
After this test, we can thus conclude that our hashBlock method works perfectly.